一、对象存储OSS
阿里云对象存储OSS(Object Storage Service)具有丰富的安全防护能力,支持服务器端加密、客户端加密、防盗链白名单、细粒度权限管控、日志审计、合规保留策略(WORM)等特性。OSS为您的云端数据安全进行全方位的保驾护航,并满足您企业数据的安全与合规要求。
二、应用场景
数据签迁移、数据湖、企业数据存储和管理、数据处理、容灾与备份

三、开通阿里云OSS
1、(1)申请阿里云账号
(2)实名认证
(3)开通“对象存储OSS”服务
(4)进入管理控制台


2、创建Bucket
选择:标准存储、公共读、不开通
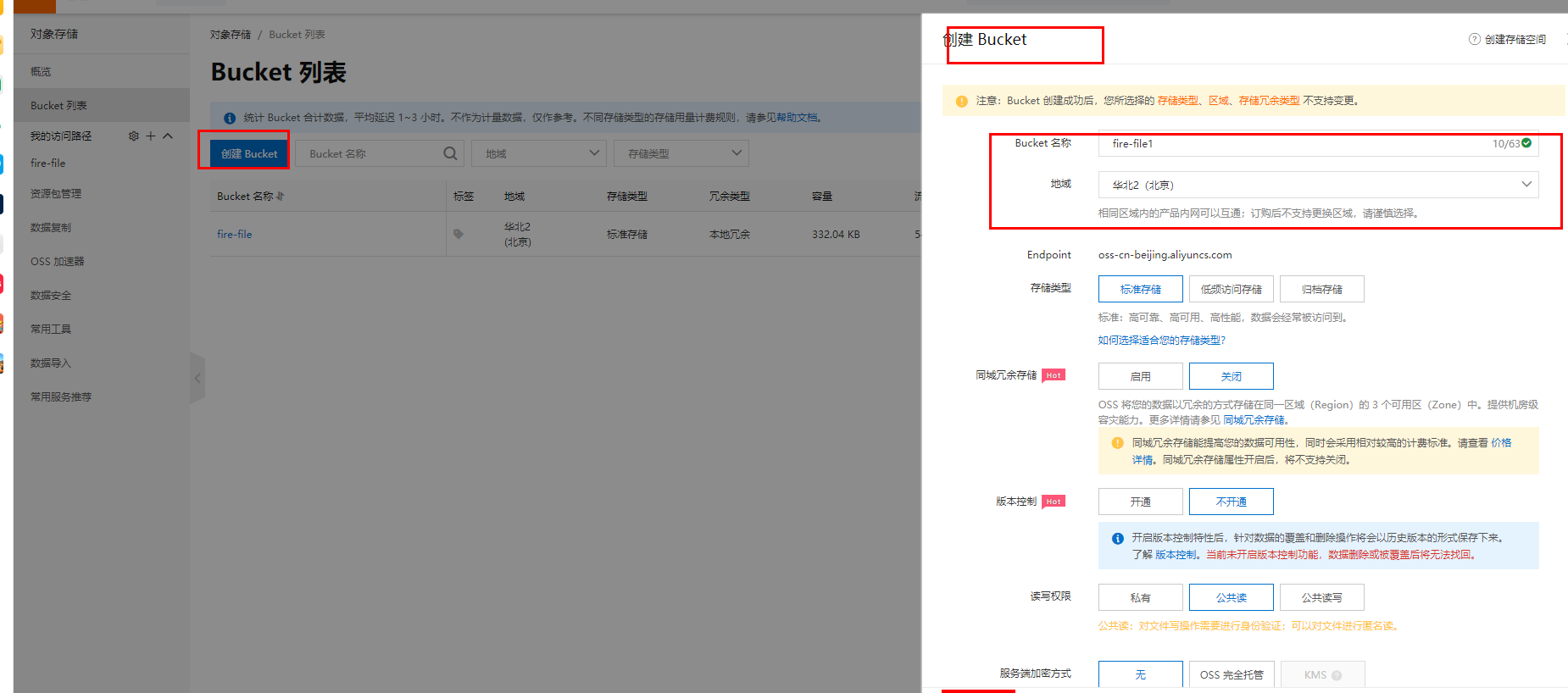
3、上传图片
创建文件夹avatar,上传默认的用户图片

4、创建RAM子用户、用于程序访问对象存储OSS

四、使用SDK

1,创建Maven项目
com.atguigu aliyun-oss
2,pom
<dependencies>
<!-- 阿里云oss依赖 -->
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
</dependency>
<!-- 日期工具栏依赖 -->
<dependency>
<groupId>joda-time</groupId>
<artifactId>joda-time</artifactId>
</dependency>
</dependencies>
3,找到编码用的常量配置
(1)endpoint (2)bucketName (3)accessKeyId (4)accessKeySecret
4,测试Bucket连接
public class OSSTest {
// Endpoint以杭州为例,其它Region请按实际情况填写。
String endpoint = "oss-cn-beijing.aliyuncs.com";
// 阿里云主账号AccessKey拥有所有API的访问权限,风险很高。强烈建议您创建并使用RAM账号进行API访问或日常运维,请登录 https://ram.console.aliyun.com 创建RAM账号。
String accessKeyId = "LTAI5tSjEbLtaqn8HMe4zF4G";
String accessKeySecret = "mGgEO1ueh5WdVK4oK4kJOSPQgHPR5m";
String bucketName = "fire-file";
@Test
public void testCreateBucket() {
// 创建OSSClient实例。
OSSClient ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
// 创建存储空间。
ossClient.createBucket(bucketName);// 关闭OSSClient。
System.out.println(ossClient.listBuckets());
// ossClient.shutdown();
}
@Test
public void testExist() {
// 创建OSSClient实例。
OSSClient ossClient = new OSSClient(endpoint, accessKeyId, accessKeySecret);
boolean exists = ossClient.doesBucketExist(bucketName);
System.out.println(exists);
// 关闭OSSClient。
ossClient.shutdown();
}
}
五、Springboot集成阿里云OSS
1,在service模块下创建子模块service-oss

2,配置Pom.xml
service-oss上级模块service已经引入service的公共依赖,所以service-oss模块只需引入阿里云oss相关依赖即可,service父模块已经引入了service-base模块,所以Swagger相关默认已经引入,上边已经引过了
3,配置Application.properties
#服务端口
server.port=8002
#服务名
spring.application.name=service-oss
#环境设置:dev、test、prod
spring.profiles.active=dev
#阿里云 OSS
#不同的服务器,地址不同
aliyun.oss.file.endpoint=oss-cn-beijing.aliyuncs.com
aliyun.oss.file.keyid=LTAI5tSjEbLtaqn8HMe4zF4G
aliyun.oss.file.keysecret=mGgEO1ueh5WdVK4oK4kJOSPQgHPR5m
#bucket可以在控制台创建,也可以使用JAVA代码创建
aliyun.oss.file.bucketname=fire-file
5,创建启动类
@ComponentScan({"com.atguigu"})
@SpringBootApplication(exclude = DataSourceAutoConfiguration.class)
public class OssApplication {
public static void main(String[] args) {
SpringApplication.run(OssApplication.class,args);
}
}
6,实现文件上传
创建常量读取工具类:
ConstantPropertiesUtil.java,使用@Value读取application.properties里的配置内容用spring的 InitializingBean 的 afterPropertiesSet 来初始化配置信息,这个方法将在所有的属性被初始化后调用。
@Component
public class ConstantPropertiesUtil implements InitializingBean {
@Value("${aliyun.oss.file.endpoint}")
private String endpoint;
@Value("${aliyun.oss.file.keyid}")
private String keyId;
@Value("${aliyun.oss.file.keysecret}")
private String keySecret;
@Value("${aliyun.oss.file.bucketname}")
private String bucketName;
public static String END_POINT;
public static String ACCESS_KEY_ID;
public static String ACCESS_KEY_SECRET;
public static String BUCKET_NAME;
@Override
public void afterPropertiesSet() throws Exception {
END_POINT = endpoint;
ACCESS_KEY_ID = keyId;
System.out.println(ACCESS_KEY_ID+"==========================");
ACCESS_KEY_SECRET = keySecret;
BUCKET_NAME = bucketName;
}
}
7,文件上传
创建Service接口:FileService.java
public interface FileService {
/**
* 文件上产到阿里云oss
* @param file
* @return
*/
String upload(MultipartFile file);
}
@Service
public class FileServiceImpl implements FileService {
@Override
public String upload(MultipartFile file) {
// 工具类获取值
String endpoint = ConstantPropertiesUtil.END_POINT;
String accessKeyId = ConstantPropertiesUtil.ACCESS_KEY_ID;
String accessKeySecret = ConstantPropertiesUtil.ACCESS_KEY_SECRET;
String bucketName = ConstantPropertiesUtil.BUCKET_NAME;
try {
// 创建OSS实例。
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
//获取上传文件输入流
InputStream inputStream = file.getInputStream();
//获取文件名称
String fileName = file.getOriginalFilename();
//1 在文件名称里面添加随机唯一的值
String uuid = UUID.randomUUID().toString().replaceAll("-","");
// yuy76t5rew01.jpg
fileName = uuid+fileName;
//2 把文件按照日期进行分类
//获取当前日期
// 2019/11/12
String datePath = new DateTime().toString("yyyy/MM/dd");
//拼接
// 2019/11/12/ewtqr313401.jpg
fileName = datePath+"/"+fileName;
//调用oss方法实现上传
//第一个参数 Bucket名称
//第二个参数 上传到oss文件路径和文件名称 aa/bb/1.jpg
//第三个参数 上传文件输入流
ossClient.putObject(bucketName,fileName , inputStream);
// 关闭OSSClient。
ossClient.shutdown();
//把上传之后文件路径返回
//需要把上传到阿里云oss路径手动拼接出来
// https://edu-guli-1010.oss-cn-beijing.aliyuncs.com/01.jpg
String url = "http://"+bucketName+"."+endpoint+"/"+fileName;
return url;
}catch(Exception e) {
e.printStackTrace();
return null;
}
}
}
控制层
@RestController
@RequestMapping("/eduoss")
@CrossOrigin
public class FileUploadController {
@Autowired
private FileService fileService;
/**
* 13
* 文件上传
* 14
* <p>
* 15
*
* @param file 16
*/
@ApiOperation(value = "文件上传")
@PostMapping("upload")
public R upload(
@ApiParam(name = "file", value = "文件", required = true) @RequestParam("file") MultipartFile file) {
String uploadUrl = fileService.upload(file);
//返回r对象
return R.ok().message("文件上传成功").data("url", uploadUrl);
}
}
重启OSS服务,用Swagger进行测试。